Your cart is currently empty!
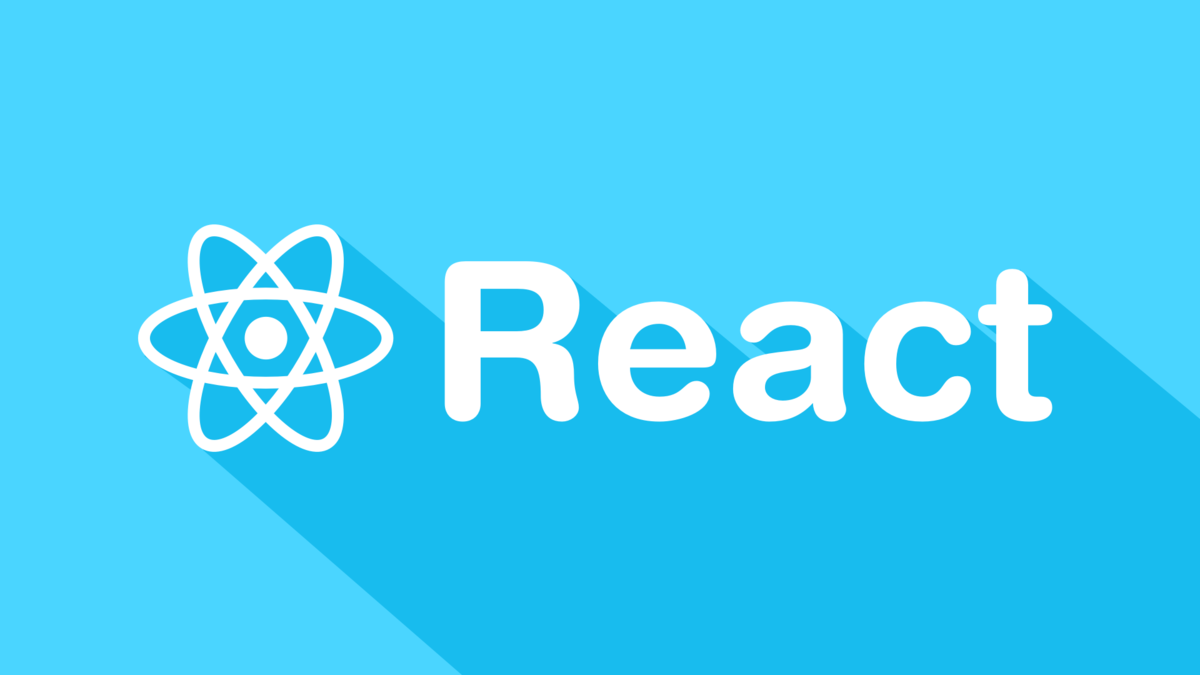
Building React Websites with Node.js and MongoDB
This documentation outlines how to develop a React website using Node.js for the backend, MongoDB for database storage, and Passport.js for user authentication. We’ll explore key areas like user management, middleware implementation, error handling, and API access.
Table of Contents
- Introduction
- Project Requirements
- Technical Overview
- Planning and Architecture
- Core Functionalities
- User Management with Sessions
- Authentication with Passport.js
- Middleware for User Roles and Rights
- Error Handling and Logging
- API Access for React Components
6. Case Study: Evaficy Smart Test
7. Conclusion
1. Introduction
This documentation serves as a roadmap for building a full-stack web application with the MERN (MongoDB, Express.js, React, Node.js) stack. It addresses essential features such as:
- Secure authentication using Passport.js.
- Role-based access control with middleware.
- API access and integration with React hooks for data flow.
A real-world example is Evaficy Smart Test (qa.evaficy.com), which combines these technologies to provide robust functionality for managing test cases and QA processes.
2. Project Requirements
Functional Requirements:
- User Authentication: Secure login/logout, session management.
- Role-Based Access: Differentiated access levels for Admin, User, and Guest roles.
- API Integration: Frontend to backend communication for CRUD operations.
- Error Management: Unified error handling and logging system.
Business Requirements:
- Scalability to handle multiple users.
- User-friendly interfaces for seamless interaction.
- Robust security measures for data protection.
3. Technical Overview
Frontend:
- React 18 with hooks for state and side effects.
- Axios for API calls.
- Component-based architecture for reusability.
Backend:
- Node.js for server-side logic.
- Express.js for routing and middleware.
- MongoDB with Mongoose for database management.
Authentication:
- Passport.js for strategies like local authentication.
- Express-session for managing session data.
Middleware:
- Role-based access control middleware.
- Error-handling middleware for consistent responses.
Deployment:
- Docker for containerization.
- Nginx for load balancing and reverse proxy.
4. Planning and Architecture
Key User Stories: Planning and Architecture
This section details the planning and architecture for the outlined user stories. Each story includes titles, descriptions, requirements, and steps of execution, ensuring clarity in the development process.
Story 1: User Registration and Login
Title: Secure User Registration and Login System
Description:
As a user, I want to register with my email and password and log in securely, so I can access my personalized dashboard. This ensures secure access to the system with robust session management and protection against unauthorized access.
Requirements:
- Frontend:
- Registration Form: Fields for email, password, and confirm password.
- Login Form: Fields for email and password.
- Validation for email format, password length, and confirmation matching.
- Error messages for incorrect credentials.
- Backend:
- API endpoints: /auth/register and /auth/login.
- Password hashing with bcrypt.
- Session management with express-session.
- Passport.js for local authentication strategy.
- MongoDB collection for storing user credentials securely.
Steps of Execution:
Frontend Development:
- Design the registration and login forms using React components.
- Implement form validation using state and hooks like useState and useEffect.
- Integrate Axios for sending user data to backend APIs.
Backend Development:
- Set up routes for /auth/register and /auth/login using Express.
- Hash passwords with bcrypt before storing them in the database.
- Configure Passport.js for local strategy and integrate it with Express-session for session persistence.
- Return appropriate responses for success, invalid credentials, or server errors.
Session Management:
- Store session details in MongoDB using connect-mongo.
- Implement middleware to check for active sessions before granting access to protected routes.
Testing:
- Validate registration with invalid inputs (e.g., weak passwords, duplicate emails).
- Test login with correct and incorrect credentials.
- Verify session persistence across browser refreshes.
Story 2: User Management and Role Assignment
Title: Role-Based User Management
Description:
As an admin, I want to manage users and assign roles to define their permissions and access levels across the system. This ensures secure operations and controlled access to resources.
Requirements:
- Frontend:
- Admin panel with a user list displaying name, email, and role.
- Dropdown or modal for assigning roles to users.
- Filter and search functionality for easy navigation.
- Backend:
- API endpoints: /admin/users (GET), /admin/users/:id/role (PATCH).
- Middleware to validate admin privileges.
- MongoDB schema for roles and permissions (e.g., Admin, User, Guest).
- Database Structure:
- Users collection: { “_id”: “user_id”, “email”: “[email protected]”, “password”: “hashed_password”, “role”: “User” }
- Roles collection: { “_id”: “role_id”, “roleName”: “Admin”, “permissions”: [“manage_users”, “view_reports”] }
- Users collection: { “_id”: “user_id”, “email”: “[email protected]”, “password”: “hashed_password”, “role”: “User” }
Steps of Execution:
Frontend Development:
- Create an admin dashboard using Material-UI or Ant Design for tables and modals.
- Use React state or a global store like Redux to manage user data.
- Fetch user data and roles from the backend API using Axios.
- Implement role assignment functionality using API calls and update the UI dynamically.
Backend Development:
- Add middleware to verify if the requesting user has admin privileges.
- Set up API endpoints to fetch user details and update their roles.
- Implement validation for role updates (e.g., prevent assigning invalid roles).
Role-Based Access Middleware:
- Define a middleware to restrict access based on roles.
- Check user roles before executing certain routes (e.g., /admin routes).
Testing:
- Test role assignment flow (Admin assigning roles to other users).
- Verify restricted actions for unauthorized roles.
- Simulate edge cases, such as assigning invalid roles or users without sufficient privileges attempting restricted actions.
Story 3: Error Logging and Diagnostics
Title: Centralized Error Handling and Logging
Description:
As a system, I should log errors to provide diagnostic information for monitoring and debugging. This ensures the stability and reliability of the application.
Requirements:
- Backend:
- Middleware to handle and log errors consistently.
- Integration with a logging library (e.g., Winston, Bunyan).
- Error logs stored in a file or sent to an external service (e.g., Logstash, ElasticSearch).
- Frontend:
- Display user-friendly error messages for API failures or invalid actions.
- Testing:
- Simulate API failures and check log entries for appropriate error information.
Steps of Execution:
Middleware Development:
- Implement an error-handling middleware in Express.
- Capture error messages and stack traces.
- Format error responses with HTTP status codes and messages.
Logging Setup:
- Configure Winston for logging errors to a file and console.
- Set up transport for error logs to external monitoring tools (e.g., Logstash).
Frontend Error Display:
- Implement a global error boundary in React for catching runtime errors.
- Display error alerts or fallback UI for failed API responses.
Testing:
- Manually trigger errors in APIs and verify log outputs.
- Test how the system recovers or redirects after critical errors.
Database Schema Design:
- Users Collection:
- Fields: username, email, hashed_password, role, sessions.
- Roles Collection:
- Fields: role_name, permissions.
- Posts Collection (if content management is needed):
- Fields: title, content, author_id, created_at.
API Endpoints:
- /auth/login: Authenticate users.
- /auth/register: Create a new user.
- /users/roles: Fetch or assign user roles.
- /posts: CRUD operations for blog posts or similar data.
Frontend-Backend Integration:
- React communicates with Express APIs for user actions.
- State management in React for session persistence.
5. Core Functionalities
User Management with Sessions
Objective: Maintain user session data securely on the server.
- Session Management: Use express-session to store session data in MongoDB.
- Session Persistence: Ensure users stay logged in across multiple requests.
Authentication with Passport.js
Objective: Authenticate users using strategies like local or OAuth.
- Password Security: Hash passwords using bcrypt for secure storage.
- Session-based Authentication: Leverage passport-local for login flows.
Middleware for User Roles and Rights
Objective: Implement role-based access control.
- Role Middleware: Define permissions for actions based on roles (e.g., Admin, User).
- Dynamic Access Control: Fetch permissions from the database to control API access.
Error Handling and Logging
Objective: Implement a centralized error-handling mechanism.
- Global Error Handler: Capture errors from middleware, controllers, and services.
- Logging: Use a logging library (e.g., Winston) to store errors in files or external services.
API Access for React Components
Objective: Enable secure data flow between React and Node.js.
- Client Communication: Axios for HTTP requests.
- React Hooks: Create custom hooks for reusable API logic.
6. Case Study: Evaficy Smart Test
Evaficy Smart Test is a QA service that generates test cases, validates them, and allows exports in JSON format. Here’s how it implements the key functionalities:
Key Stories and Execution:
1. User Authentication:
- Users log in to access personalized dashboards.
- Admins manage user roles and test case validation.
2. API Integration:
- CRUD operations for test cases with proper role validation.
- JSON export feature for test case sharing.
3. Error Handling:
- All errors are logged to a centralized system.
- Custom error pages for better user experience.
Workflow:
- Users register and authenticate via Passport.js.
- Middleware checks permissions before granting API access.
- Frontend React hooks manage data flow, updating UI dynamically.
7. Our Conclusion
This documentation outlines a step-by-step approach to building a scalable and secure web application using React, Node.js, MongoDB, and Passport.js. By integrating these tools effectively, developers can create feature-rich applications with user roles, session management, and robust error handling. The example of Evaficy Smart Test demonstrates practical implementation, making this a valuable resource for developers and businesses alike.